Description
Transient stores are used as repositories for transient (automatic) data. Each store contains a configurable number of buffers, and readers and writers are blocked if the store is empty or full respectively. Reads are destructive, but the data is conserved until the last sharing reader completes (see Distributors). TST data therefore provides an equivalent to stack data (persists while referenced).
The read/write cycle is described in more detail in the connective logic overview (see Connective Logic Overview).
Creation Attributes
Name
Instance name (see Object Names)
Dimensions
Instance dimensionality (see Object Dimensionality)
Presence
Instance presence flag (see Object Presence)
Buffer Depth
This attribute determines the number of buffers owned by the store. If the store is single buffered then it can only be opened simultaneously by one reader or one writer. If the store has multiple buffers then each of these can be simultaneously open for either read or write. Typically, stores are created with two buffers because this allows one reader to access the data, while a separate writer is populating the other buffer.
Buffer Allocation
This attribute determines the buffer storage mode used by the store. Transient stores support four modes. In 'static' mode, buffers are created when the store is created and their size cannot change. In 'extendible' mode, buffers are re-allocated if their constructed size is greater then their existing size. In 'conforming' mode, buffers are re-allocated if their constructed size differs from their existing size. In 'dynamic' mode, buffers are created when opened for write, and deleted when closed after read. If the data is fixed size then 'static' mode is preferable. If the data is variable sized then extendible mode will have less CPU overhead but require more memory to be allocated. If memory is at a premium, then the 'dynamic' mode will use the least memory. By default, the allocation mode will be 'conforming'.
Buffer Size
This attribute determines the initial buffer size at creation time. If the store uses 'static' buffer allocation, then this size will be fixed. If the store uses 'dynamic' memory allocation then the initial size should be zero.
Data Affinity
This attribute determines whether or not buffers that are constructed by slaved methods are flushed back to the master on closure. If this flag is set to 'ENDPOINT' then data will always be flushed back. If it is set to 'FLOATPOINT' then the data stays in the slave until it is required as input to some later calculation, and is then moved directly to the requesting slave. Endpoint data is safer but floatpoint data requires less network bandwidth. Floatpoint data cannot be accessed by the master.
Record Type
This attributes identifies the record type that the store holds (see store records).
Connection Attributes
Transient store connections require the following attributes;
Timeout
This attribute determines whether the connection will poll or block (see Connection Timeout).
Signature
This attribute is a string that describes the mapping from elements in the consumer, to elements in the transient store. See Connection Mappings.
Dimensions
This attribute determines the dimensionality of the connection array owned by each consuming element. By default, this will provide the consuming element with one connection for each provider element. Collector, Multiplexor and Manual connection consumers can have multi-dimensional connection arrays, but in all other cases, this attribute will be '1'. See Connection Mappings.
Repeat Count
This attribute determines the number of times that each 'provider element to consumer element' connection is repeated. If the repeat count is greater then one, then the Dimensions attribute (above), and the resulting connection access functions, will both have an 'extra' dimension. Only Collector, Multiplexor and Manual connection consumers can have repeat counts that are greater than '1'. See Connection Mappings.
Name
This attribute determines the connection's 'name' which is used to construct access function names (see Connection Names).
Access
This attribute determines whether the consumer is requesting read or write access to the store's data.
Key
This attribute can be used as a means of filtering reads. If a writer specifies a key then it is associated with the buffer instance. Readers that specify a key and a rule will only be granted access to data that satisfies their specified constraint. See Store Keys and Rules.
Rule
This attribute can be used by readers as a means of filtering out data of interest (see Store Keys and Rules).
Additional attributes may also be required that are specific to the connection's consuming object. These are documented in their respective sections.
Manual Connections
Manual connections provide a means of directly accessing target objects. These are created automatically from CDL diagrams and accessed using their access function names (see Manual Connections).
Arbitrated store connections provide the following member functions;
OpenWrite()
This member function opens the store for write access (see TST OpenWrite function).
OpenRead()
This member function opens the store for read access (see TST OpenRead function).
Close()
This member function closes a store that was successfully opened for write or read (see TST Close function).
Automatic and Manual Connections
The following member functions can be used with both automatic and manual connections;
AbortWrite()
This member function aborts a write to a store that has been successfully opened for write, but not yet closed. The 'close' call is still required. See TST Abort function.
AbortReadToBack()
This member function aborts a read from a store that has been successfully opened for read, but not yet closed. The 'close' call is still required. See TST AbortToBack function. The read buffer is returned to back of the store's readable buffer queue where it can be read again at a later stage.
AbortReadToFront()
This member function aborts a read from a store that has been successfully opened for read, but not yet closed. The 'close' call is still required. See TST AbortToFront function. The read buffer is returned to the front of the store's readable buffer queue where it can be read again at a later stage. Note that buffers returned to the front of the queue will be the 'next' buffers read.
Record()
If the connection has been successfully opened, and not yet closed, then this member function returns a reference to the store's record object. If the buffer mode (see above) is not 'static', and the store has been opened for write access, then the data object will need to be constructed before it can be referenced (see access functions and store records).
IsOpen()
This member function returns TRUE if the connection is 'Open' or FALSE otherwise.
Key()
This member function returns the key that is associated with the currently 'Open' buffer (see key attribute above).
Notes
There are a number of points to bear in mind when using transient stores;
Store data object alignment is 32 byte. This can be important for some high performance math libraries that require data to be aligned. It is sometimes necessary therefore to 'pad' data objects.
It is possible to create deadlocks when accessing two or more stores in inconsistent order, but in practice multiple store access is usually handled by collectors and so deadlocks are extremely unlikely to occur, and are completely avoidable (see example below).
Distributor objects provide a simple means of sharing store access, but shared writes are only permitted when the store and its writers are executing in the same process.
It is sometimes necessary to initialize stores to contain readable data prior to starting main process execution. This is typically achieved through use of manual connections as part of user circuit initialization code.
Readable events are generated in the order in which they were closed for write, rather than the order that they were opened for write.
Examples
The example below shows a private store named 'Tst', with data type 'Dtype', and dimensionality 'NxM'.
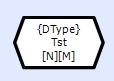